create custom analog clock widget in this tutorial.
Widget.java
package sandy.Tutorials.Widgets.AnalogClock;
import android.app.PendingIntent;
import android.appwidget.AppWidgetManager;
import android.appwidget.AppWidgetProvider;
import android.content.ComponentName;
import android.content.Context;
import android.content.Intent;
import android.widget.RemoteViews;
public class Widget extends AppWidgetProvider
{
public void onReceive(Context context, Intent intent)
{
String action = intent.getAction();
if (AppWidgetManager.ACTION_APPWIDGET_UPDATE.equals(action))
{
RemoteViews views = new RemoteViews(context.getPackageName(), R.layout.widget);
Intent AlarmClockIntent = new Intent(Intent.ACTION_MAIN).addCategory(Intent.CATEGORY_LAUNCHER).setComponent(new ComponentName("com.android.alarmclock", "com.android.alarmclock.AlarmClock"));
PendingIntent pendingIntent = PendingIntent.getActivity(context, 0, AlarmClockIntent, 0);
views.setOnClickPendingIntent(R.id.Widget, pendingIntent);
AppWidgetManager.getInstance(context).updateAppWidget(intent.getIntArrayExtra(AppWidgetManager.EXTRA_APPWIDGET_IDS), views);
}
}
}
Info.java
package sandy.Tutorials.Widgets.AnalogClock;
import android.app.Activity;
import android.os.Bundle;
public class Info extends Activity
{
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState); setContentView(R.layout.info);
}
}
widget.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/Widget"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:gravity="center" >
<AnalogClock
android:id="@+id/AnalogClock"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:dial="@drawable/widgetdial"
android:hand_hour="@drawable/widgethour"
android:hand_minute="@drawable/widgetminute"
/>
</RelativeLayout>
info.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Thank you for installing the Custom Analog Clock Widget.\n\nPlease read these important instructions.\n\nThis is a widget, not a regular application. Unlike regular applications, widgets are placed on the home screen and offer useful (hopefully) information at a glance. In many cases, such as with this widget, you can press the widget and view additional, or more detailed, information. Pressing this widget will open the Alarm Clock application.\n" />
</LinearLayout>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="sandy.Tutorials.Widgets.AnalogClock" android:versionCode="1" android:versionName="1.0">
<application android:icon="@drawable/icon" android:label="@string/app_name">
<activity android:name=".Info" android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.INFO" />
</intent-filter>
</activity>
<receiver android:name=".Widget" android:label="Custom Analog Clock">
<intent-filter>
<action android:name="android.appwidget.action.APPWIDGET_UPDATE" />
</intent-filter>
<meta-data android:name="android.appwidget.provider" android:resource="@xml/widget" />
</receiver>
</application>
<uses-sdk android:minSdkVersion="3" />
</manifest>
following images used for analog clock

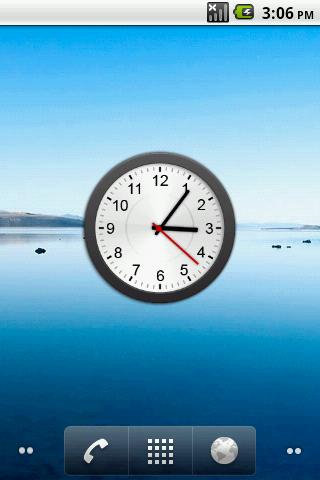
UI when you run this tutorial
for Source code click here to
Download.
Thanks for the Tutorial!! It worked Great. 1 question. what would it take to make it sizable?
ReplyDeleteHi,how to make second needle rotate in analog clock
ReplyDeleteFix download link please!
ReplyDeleteCan I use these images for my own work?
ReplyDeleteThanks
Sanjiv
Fix download link please!
ReplyDeletefix download link pls
ReplyDeletecan you re add it so we can download it please? :)
ReplyDeletePretty please
ReplyDeleteGreat help!!Thanks for post
ReplyDeleteThis comment has been removed by the author.
ReplyDelete